Buat CRUD Di Django Dengan Class Based View
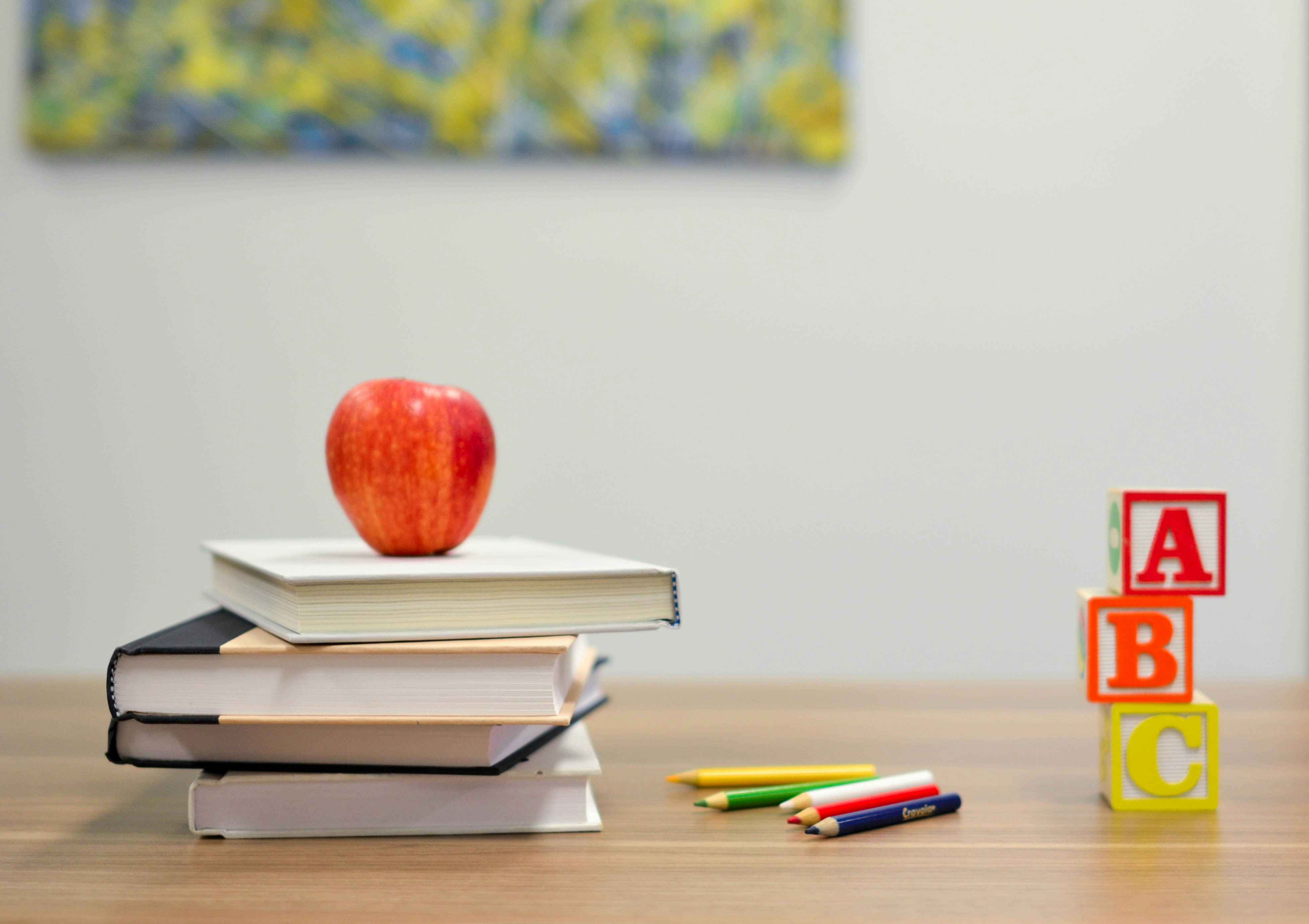
What are class-based views anyway?
Django's class-based generic views provide abstract classes implementing common web development tasks. These are very powerful, and heavily-utilise Python's object orientation and multiple inheritance in order to be extensible. This means they're more than just a couple of generic shortcuts — they provide utilities which can be mixed into the much more complex views that you write yourself.
Sumber = Django Official , CCBV
> Buat Enviroment Nya Terlebih Dahulu, Dan Install Django
Sumber = Django Official , CCBV
> Buat Enviroment Nya Terlebih Dahulu, Dan Install Django
> Buat Project Nya Dan Masuk Ke Directori
$ django-admin startproject main
$ cd main
> Buat Apps Pada Project
$ python manage.py startapp crud
#Tambahkan Pada Halaman Settings, main/main/settings.py
INSTALLED_APPS = [ ...... 'crud', ...... ]
TEMPLATES = [ { ..... 'DIRS': ['views'] ..... } ]
> Buat Models Untuk Data Crud, main/crud/models.py
models.py*
from django.db import models class DataCrud(models.Model): nama = models.CharField(max_length=200) umur = models.IntegerField() created_at = models.DateTimeField(auto_now_add=True)
> Migrations Models
$ python manage.py makemigrations
$ python manage.py migrate
> Buat Direktori Views Di Dalam Folder Project Dan File HTML
# Simpan File HTML, main/views/
main |__ crud |__ main |__ views |__ create.html |__ delete.html |__ list.html |__ update.html
> Pada File views.py, main/crud/views.py
views.py*
from django.shortcuts import render from django.views.generic import ( ListView, CreateView, UpdateView, DeleteView ) from crud.models import DataCrud class ListMain(ListView): template_name = 'list.html' model = DataCrud class CreateMain(CreateView): template_name = 'create.html' model = DataCrud fields = ['nama','umur'] success_url = '/' class UpdateMain(UpdateView): template_name = 'update.html' model = DataCrud fields = ['nama','umur'] success_url = '/' class DeleteMain(DeleteView): template_name = 'delete.html' model = DataCrud success_url = '/'
template_name = Memberitahu Class, halaman yang html yang di gunakan
model = Memberitahu, Data atau Models mana yang kita gunakan
success_url = Digunakan Saat Proses Berjalan Dan Sukses Akan Di Redirect Kehalaman Utama atau /
> Pada File urls.py Di Folder crud, Buat Jika Belum Ada, main/crud/urls.py
urls.py*
from django.urls import path from crud.views import ( ListMain, CreateMain, UpdateMain, DeleteMain, ) urlpatterns = [ path('',ListMain.as_view()), path('create',CreateMain.as_view()), path('update/<int:pk>',UpdateMain.as_view()), path('delete/<int:pk>',DeleteMain.as_view()), ]
> Pada File urls.py Di Folder main, main/main/urls.py
urls.py*
from django.contrib import admin from django.urls import path,include urlpatterns = [ path('admin/', admin.site.urls), path('',include('crud.urls')), ]
> Pada File HTML Di Folder Views, main/views/*html
list.html*
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title></title> <style> table { font-family: arial, sans-serif; border-collapse: collapse; width: 100%; } td, th { border: 1px solid #dddddd; text-align: left; padding: 8px; } tr:nth-child(even) { background-color: #dddddd; } </style> </head> <body> <a href="/create">Tambah Data</a> <table> <tr> <th>Nama</th> <th>Umur</th> <th>Update</th> <th>Delete</th> </tr> {% for obj in object_list %} <tr> <td>{{obj.nama}}</td> <td>{{obj.umur}}</td> <td><a href="/update/{{obj.id}}">Update</a></td> <td><a href="/delete/{{obj.id}}">Delete</a></td> </tr> {% endfor %} </table> </body> </html>
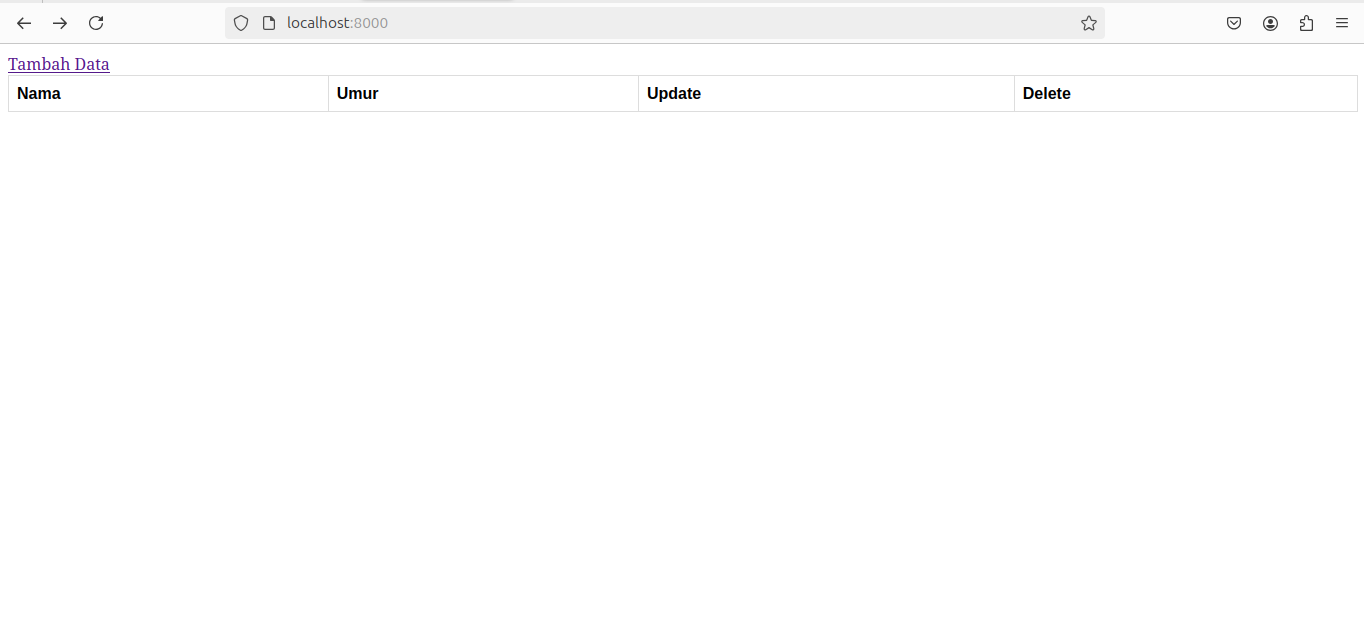
create.html*
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title></title> </head> <body> <form method="POST"> {% csrf_token %} {{form}} <input type="submit" name="Create"> </form> </body> </html>
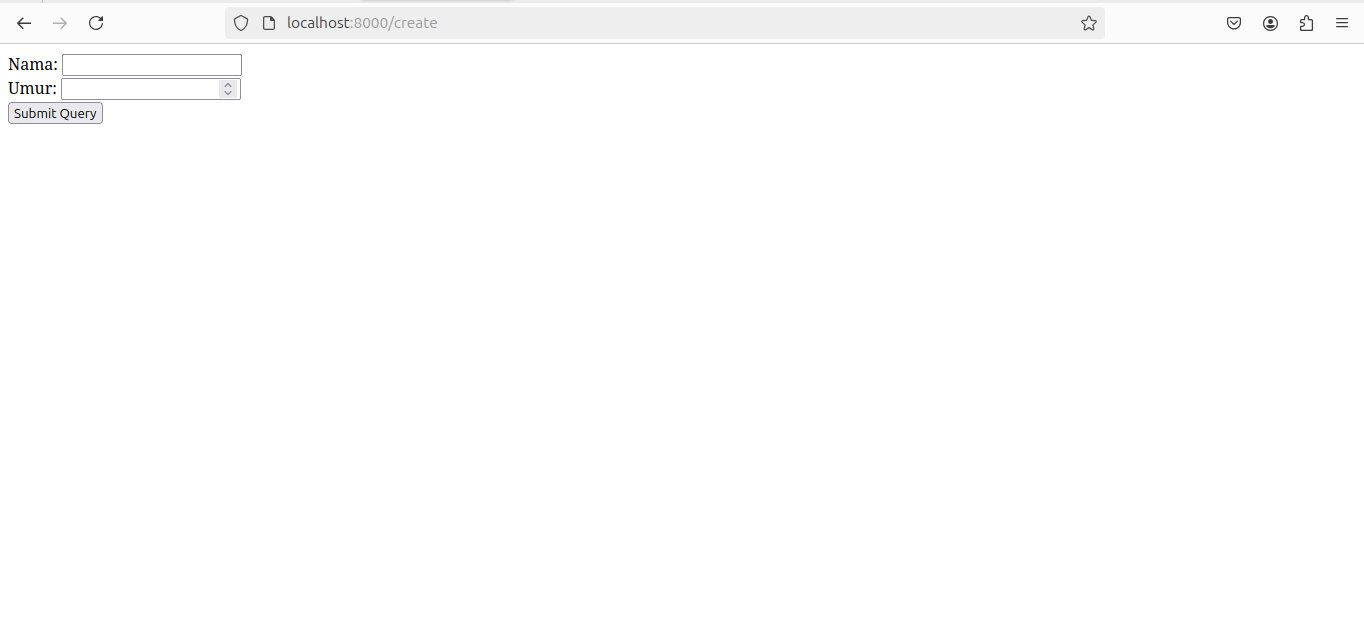
update.html*
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title></title> </head> <body> <form method="POST"> {% csrf_token %} {{form}} <input type="submit" name="Update"> </form> </body> </html>
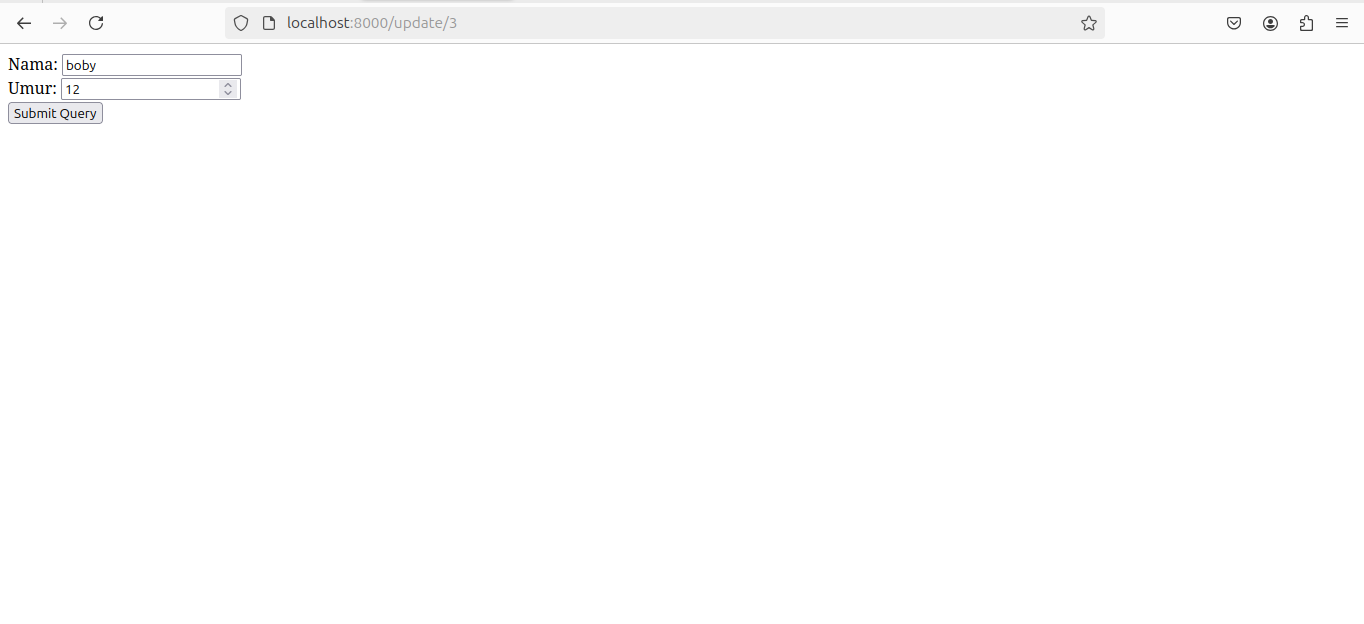
delete.html*
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title></title> </head> <body> <h2>Apakah Anda Yakin Ingin Menghapus <b>{{object.nama}}</b></h2> <form method="POST"> {% csrf_token %} <button type="submit">Yes</button> </form> <a href ="/"><button>Cancel</button></a> </body> </html>
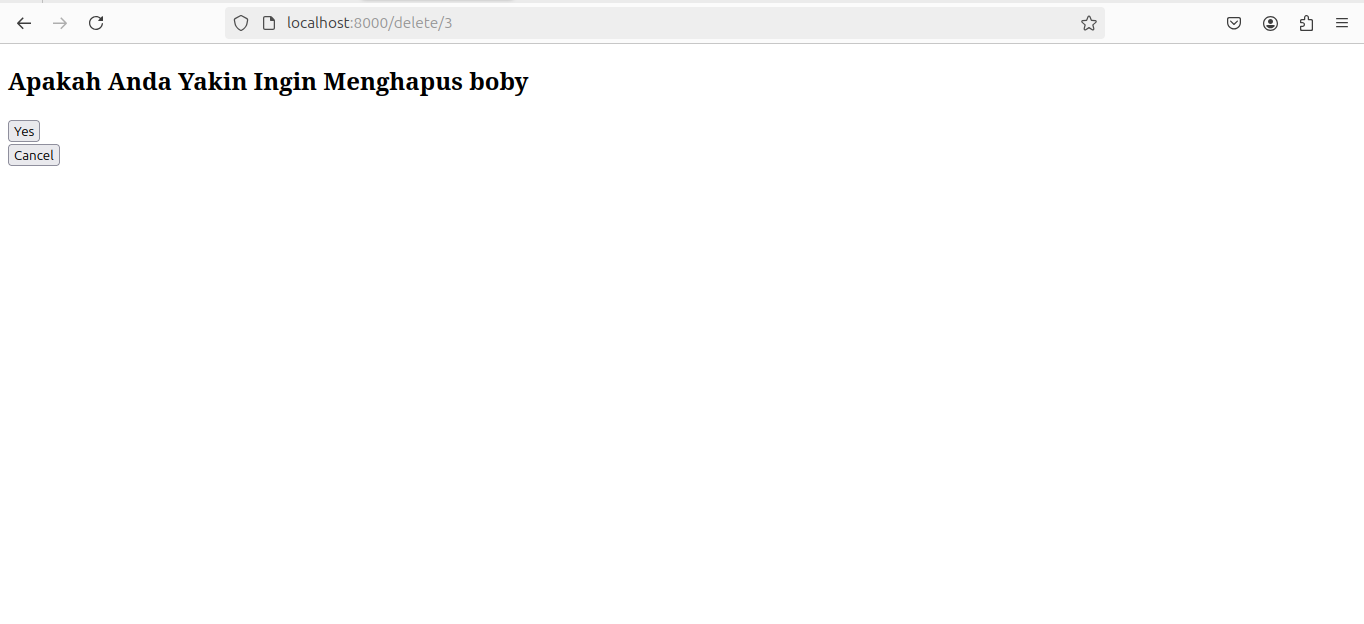
> Jalankan Server
$ python manage.py runserver
Done..... Try It
Sumber Materi